Web Development & Programming
Unit 2 Lab 2
JavaScript Methods
Mr. Torres, Instructor
Learning Outcomes
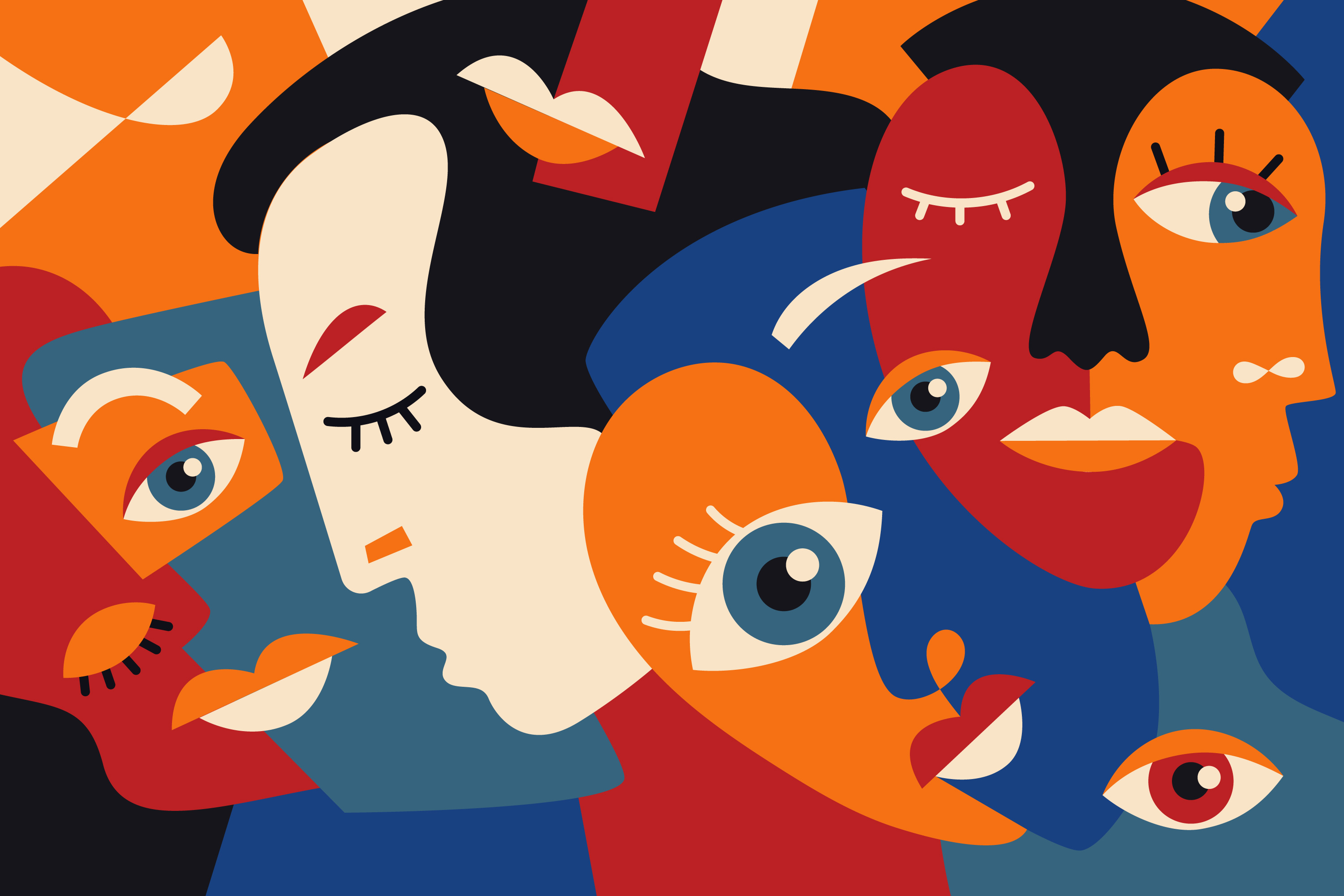
Abstraction
I can define abstraction and explain its importance in defining and using functions in JS
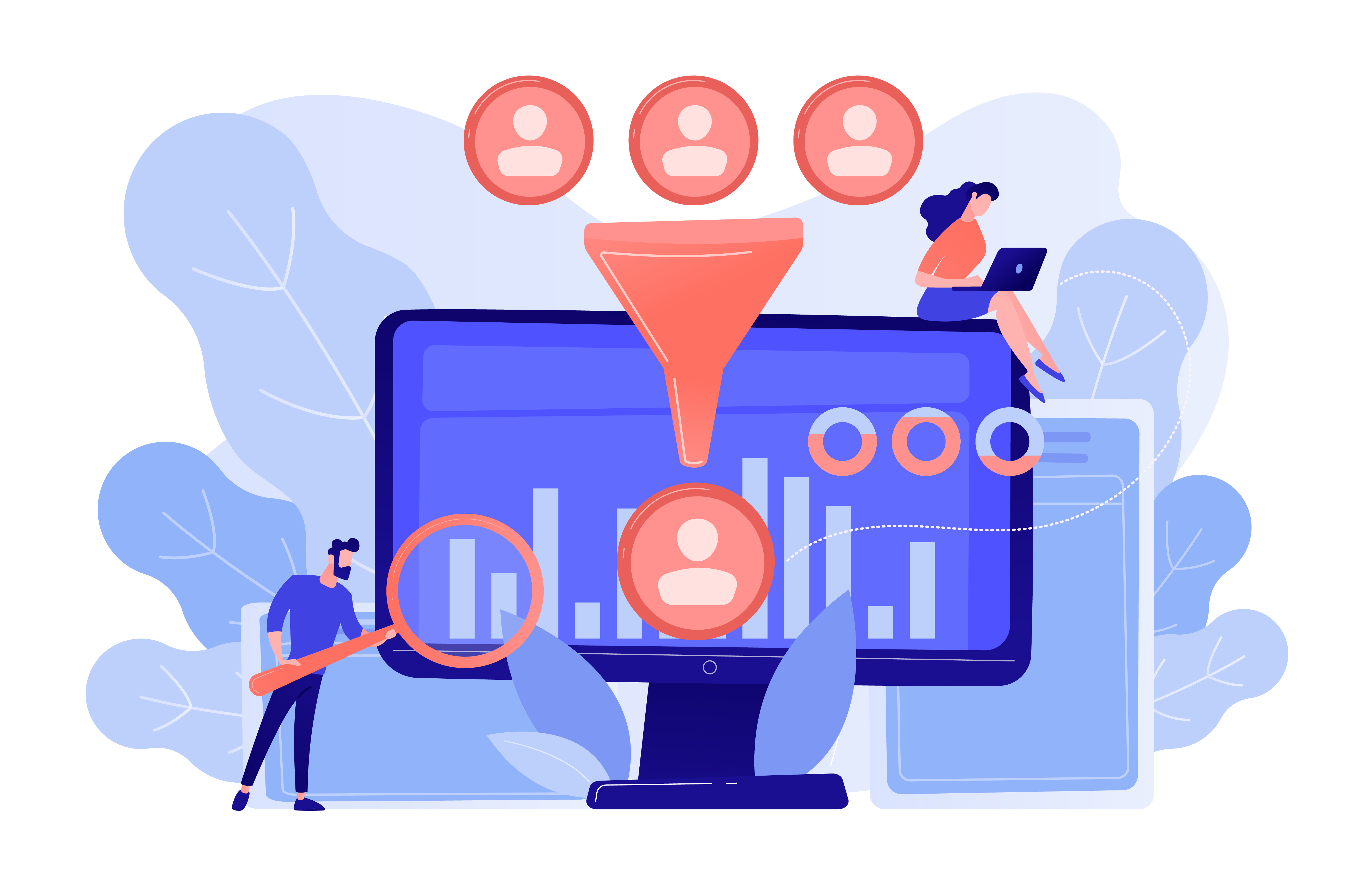
Parameters
I can write JS objects that contain and use methods while using appropriate syntax

Debugging
I can use various approaches to identify, test, and correct errors in my code.
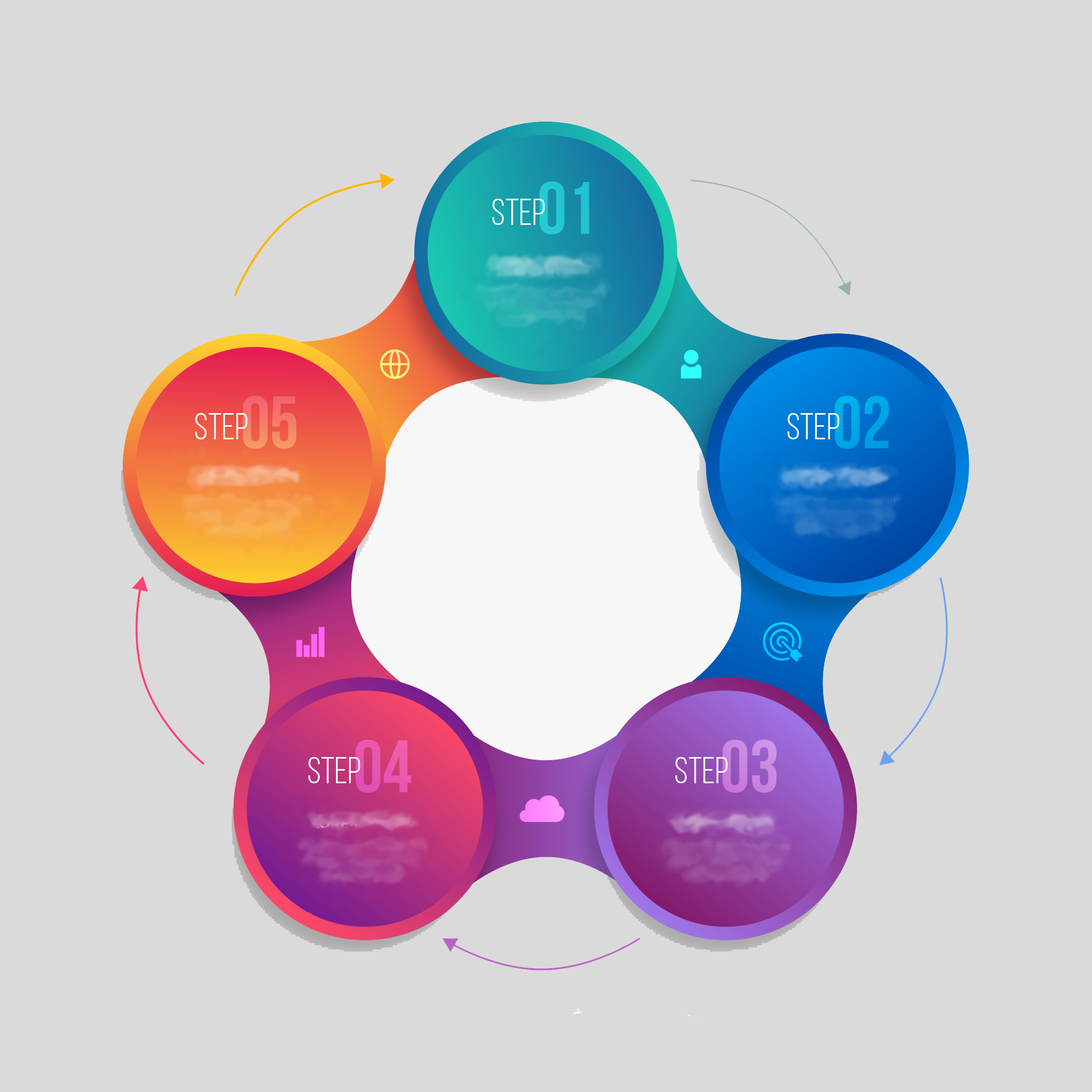
Nesting
I use function calls (and their returned values) as arguments for other functions.
Focus Question: How do we define and call functions in JS?
Workspace Configuration
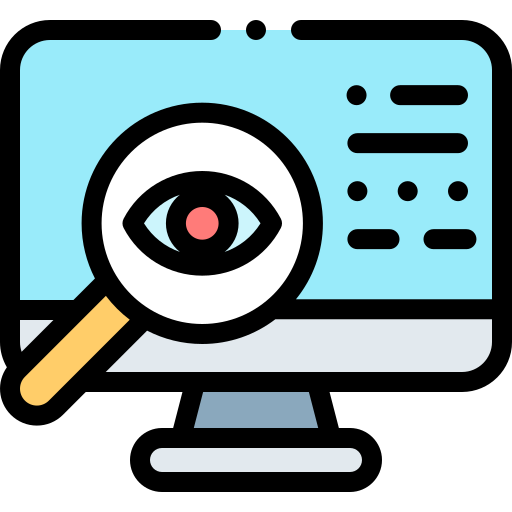
Coding Monitor (L)
Please open VS Code. Open a new folder and name it "U2L2". In that folder create and link the following files:
- index.html
- style.css
- script.js
- bootstrap.css
- bootstrap.js
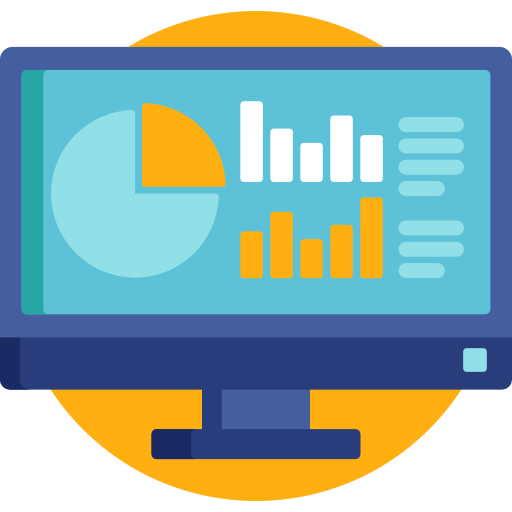
Support Monitor (R)
Please open a Google Chrome window open the following tabs:
- A Google Classroom tab (please login & go to our class page)
- A W3Schools tab (no login)
- A Bootstrap tab (no login)
- A Google search tab (no login)
- A YouTube tab (no login)
SPECIAL NOTE: you may need to download the bootstrap libraries to your computer, then upload it to your folder
JavaScript Functions
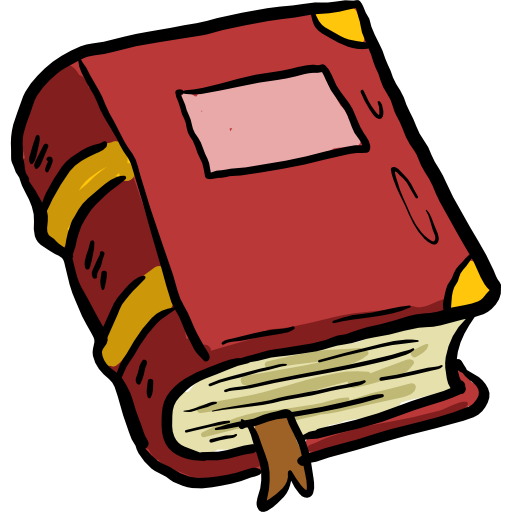
What is a JavaScript function?
- A. Let's begin by reading documentation to gain a general understanding of JavaScript Functions. Here's what you need to do:
- Read through the documentation on this page.
- Make notes as you read so that you are able to answer the questions in the next task.
-
B. Next you will demonstrate
your understanding of the text by responding to some questions based on
the reading. Complete the following tasks:
- Make a copy of the U2L3 Workbook.
- Go to the written responses tab and respond to all prompts.
Audio/Visual Learning
- A. Now, let's enhance our learning by watching JS functions at work in live videos. Here's what you need to do:
- Set up your notes so that you can continue to build a reference as you deepen your understanding.
- Watch the video linked here (also embedded below).
JavaScript Function Definitions
- A. The code snippet below shows how to define a basic javascript function. In this type of JS function there is no data entering the function using parameters (and arguments), nor is any data being returned by the function. The function will only work with what is defined in the function.
- B. The function will only work with what is defined inside the function.
-
function quizQuest(){ const userAns = prompt("What is the capital of the United States?"); if(users == "Washington, D.C."){ console.log("You are correct"); } else { console.log("You are wrong"); }
Application: Basic Functions
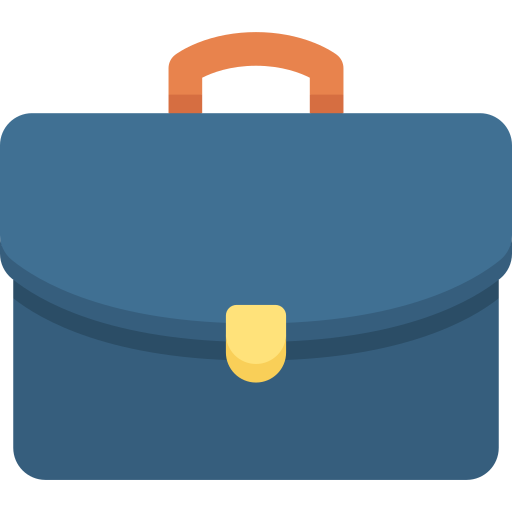
- Go to the "Problem Sets" page and complete Problem Set 1 .
What are functions with parameters?
- A. You have already learned about parameters and arguments. However, let's review them a bit more to deepen our understanding. Here's what you need to do:
- Read through the documentation on this page.
- Make notes as you read so that you are able to answer the questions in the next task.
Audio/Visual Learning
- A. Now, let's enhance our learning by watching JS functions at work in live videos. Here's what you need to do:
- Set up your notes so that you can continue to build a reference as you deepen your understanding.
- Watch the video linked here (also embedded below).
JavaScript Parameters & Arguments
- A. The code snippet below shows how to define a javascript function that allows data to enter the function (and be used) using parameters. Parameters are local variables that hold data that is given to the function when the function is called. The value(s) given when the function is called are known as arguments.
- B. In this function, username is the parameter name and is passed through (used in specific parts of the function) twice inside the function. The argument given to the function when called, is "bobby".
-
function quizQuest(username){ const userAns = prompt("What is the capital of the United States?"); if(users == "Washington, D.C."){ console.log(`You are correct, ${username}`); } else { console.log(`Sorry, ${username}, You are wrong`); }
Application: Functions with Parameters
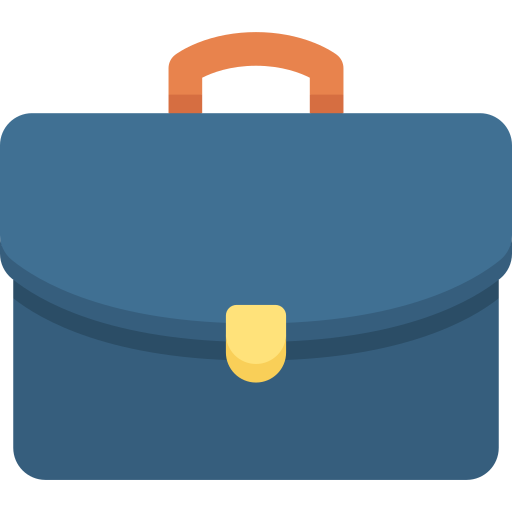
- Go to the "Problem Sets" page and complete Problem Set 2 .
Return Statements
- A. Let's look into how to get data out of a function using return statements. Here's what you need to do:
- Read through the documentation on this page.
- Make notes as ou read so that ou are able to better understand how the return statement works and why we need to use it.
Audio/Visual Learning
- A. Now, let's enhance our learning by watching live demos on using return statements in JS. Here's what you need to do:
- Set up your notes so that you can continue to build a reference as you deepen your understanding.
- Watch the video linked here (also embedded below).
- A. The code snippet below shows how to define a function that returns a value. The return statement makes the function call equal to whatever the function returns. This means ou can use the returned value for some other purpose (i.e. as the argument for another function, etc.) in our program.
- B. It is important to note that ABSOLUTELY no code will be executed AFTER the return statement executes.
-
function quizQuest(username){ let response; const userAns = prompt("What is the capital of the United States?"); if(userAns === "Washington, D.C."){ return `You are correct, ${username}`; } else{ return `Sorry, ${username}, you are wrong!`; } } quizQuest("Abdul");
SUMMARY
- Functions have a very specific syntax and begin with the keyword "function".
- Functions can be defined with or without parameters.
- Parameters are local variables to the function that hold whatever argument values they are given. .
- We call a function (or make it run its code) by using its name, followed by parentheses (with or without parameters).
Workbook Tasks: U2L2
- Open your U2L2 Workbook and respond to the question prompts on JS Functions.
-
- Be sure to write your responses in your own words
- Write thorough, thoughtful responses that address the prompts
ADDITIONAL RESOURCES: JavaScript Functions
-
Websites
Here are some more articles to look into...
-
Videos
Here are some additional videos to check out...
Problem Sets: Knowledge Application
NOTES ON chatGPT, AI Bots, and Plagiarism
- In this course, chatGPT, novaAI, and other AI bots are amazing new technologies that we can use to learn from. They should be employed as such. It is unethical to use code generated by AI bots, taken from other people, or from other sources and claim that it is yours.
- The work that you submit must represent your own effort.
- You may borrow code from others (including AI bots) sparingly and only when necessary. In these sitautions, you must give attribution (i.e. cite your code)
- All plagiarized work will receive a failing grade.
- No credit will be awarded for any code submitted that you cannot fully explain in an question and answer session with your teacher——regardless of commenting on your work.
- Your teacher reserves the right to refuse to accept, evaluate, or review any work that has been proven to be plagiarized according to any of the means listed above.
NOTES ON YOUR CODE DEVELOPMENT
- A. Use a flowchart to guide your scripting process. Here are a few things to keep in mind as you code your happy heart out:
- Your code should be completely abstracted using modularization
- The program interaction should be either in the console or via dialogue boxes using prompt( ), confirm( ), alert( ), etc. when applicable
- Use the console to "see" what the program is doing
- DEBUG Your code as you go along (do not wait until the very end)
- Use the S.N.O.T protocol to support your progress
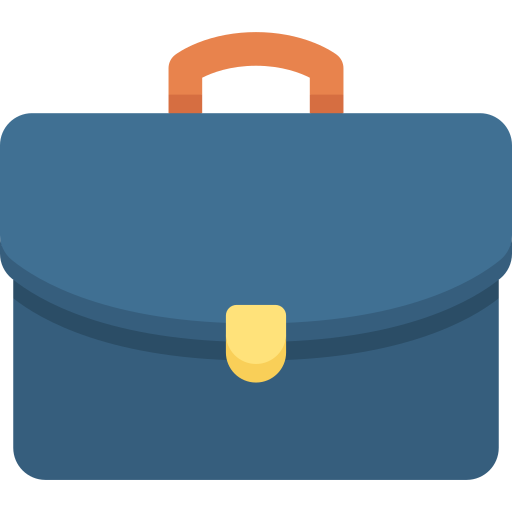
Problem Set 1: Basic JS Functions
Directions: Use your knowledge of JS function syntax to complete the problems below
Problem 1: Define Functions w/o Parameters
- A. Define a function named math. This function will:
- ask the user for two numbers (not strings) and a math operation (+, -, *, /).
- calculate the answer and alert (use the alert() ) the entire problem along with the answer.
- Debug your code.
- Finally, call the function.
Problem 2: Define Functions w/o Parameters
- B. Define a function named access. This function will:
- ask the user for their username and their password.
- print the user name and the password in an alert (use the alert() ).
- Debug your code.
- Finally, call the function.
Problem 3: Define Functions w/o Parameters
- C. Define a function named number_picker. This function will:
- ask the user to pick a number.
- if the number is greater than 50, tell them they won the beauty pageant in an alert.
- if the number is less than 50, tell them they won the lottery in an alert.
- if the number is exactly 50, tell them that they lost.
- Debug your code.
- Finally, call the function.
Problem Set 2: Functions with Parameters
Directions: Use your knowledge of JS functions to complete the problems below. Remember that these problems will require arguments.
Problem 4: Define Functions w/ Parameters
- A. Define a function named favorite_foods. This function will:
- take in a list (array) of the user's favorite foods.
- alert each item in order from most favorite to least favorite, one after the other ( use the alert() )
- Debug your code.
- Finally, call the function with a list as an argument.
Problem 5: Define functions w/ Parameters
- B. Define a function named driverLicense. This function will:
- take in the user's age, if they passed the driver's license test (true or false value) and if they want their license.
- if the user is 16 or older, passed their road test, and want their license, alert "your license has been granted"
- if one or more conditions is not met, then inform them they cannot get their license.
- inform the the user of the condition or conditions they did not meet.
- Debug your code.
- Finally, call the function.
Problem 6: Define Functions with Return Statements
- A. Define a function named favorite_quote. This function will:
- take in a favorite quote and a number.
- add the quote the number of times of the parameter to an empty array.
- return the array.
- alert the array using the alert().
- debug your code.
- Call the function.
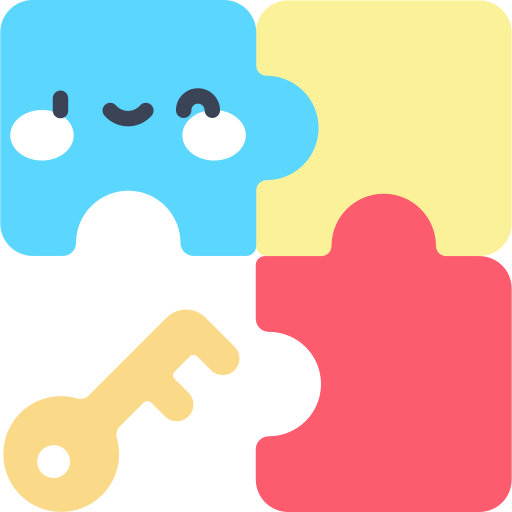
Challenge Problems: JS Functions
Directions: Use your knowledge of JS functions to complete the problems below.
Problem 7: Define Functions with Return Statements
- B. Define a function named number_rank. This function will:
- take in two numbers.
- determine which number is larger than the other.
- return a statement saying which number is greater (example: "54 is greater than 12").
- debug your code.
- Call the function.
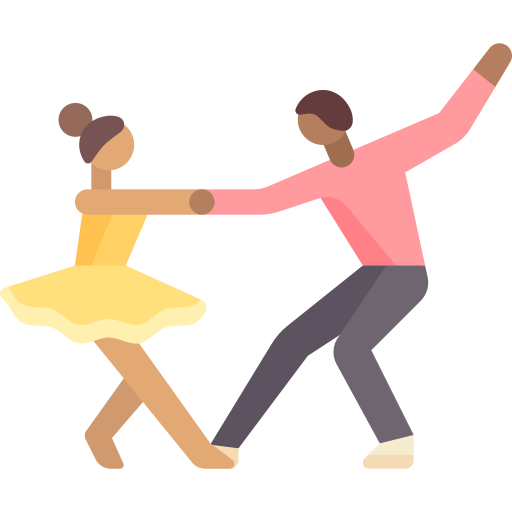
Methods
a method is a function which is a property of an object. There are two kinds of methods: instance methods or static methods.
Learn More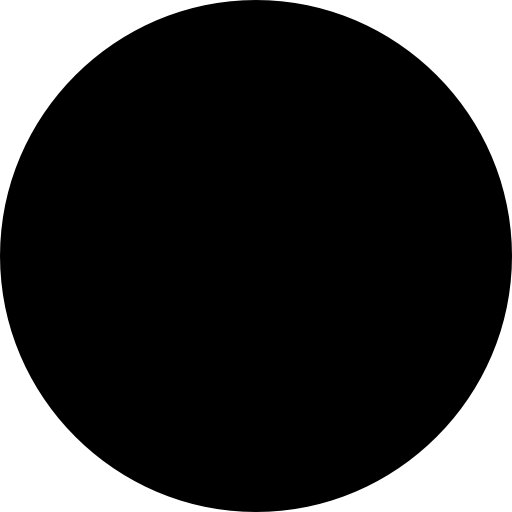
Dot Notation
a way to access a property of an object. To use dot notation, write the object name, followed by a dot(.), followed by the property name.
Learn More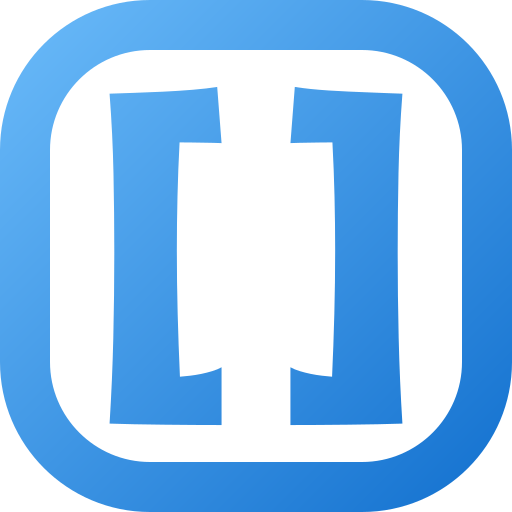
Bracket Notation
a way to access a property of an object. To use it notation, write the object name, followed by brackets [] . Inside the brackets, write the property name as a string.
Learn More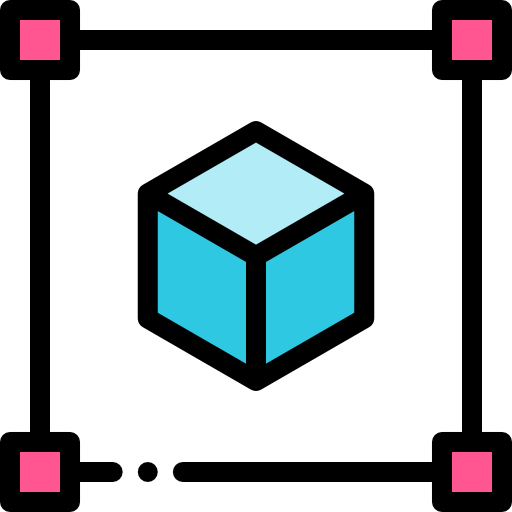
Objects
an object is an unordered collection of key-value pairs. Note, at times you will see the term "name-value" pairs instead of key-value pairs.
Learn More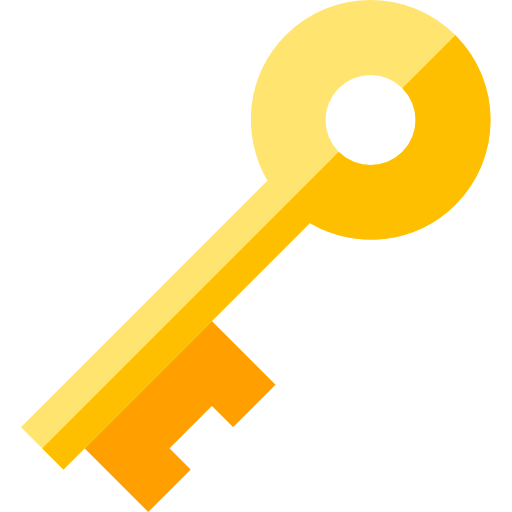
Keys
the name given to the properties contained inside an object. These keys are to be paired with a value. Keys can be strings or non-strings.
Learn More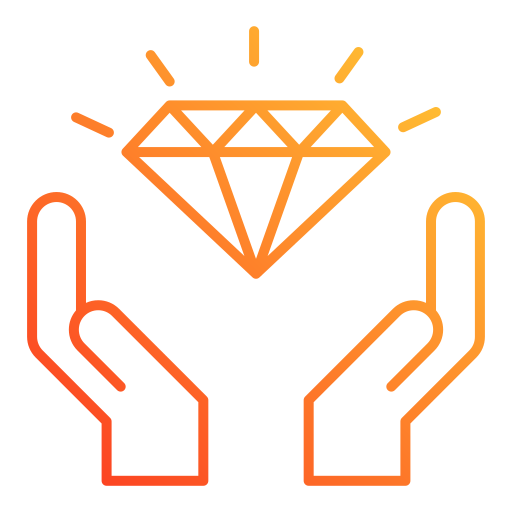
Values
the data associated (assigned) to a key in a javascript object. These values are to be paired with a key in a javascript object.
Learn More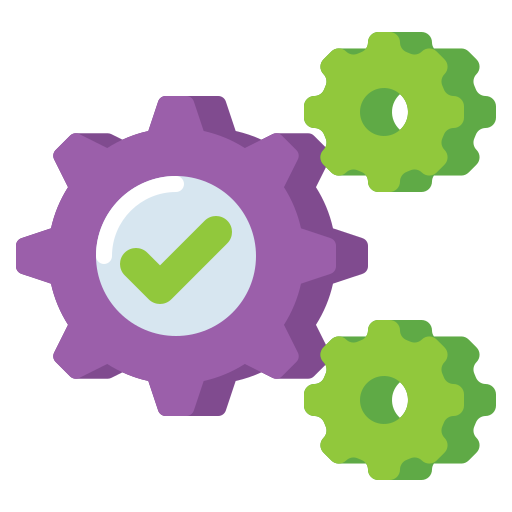
Functions
A function is simply a “chunk” of code that you can use over and over again, rather than writing it out multiple times.
Learn More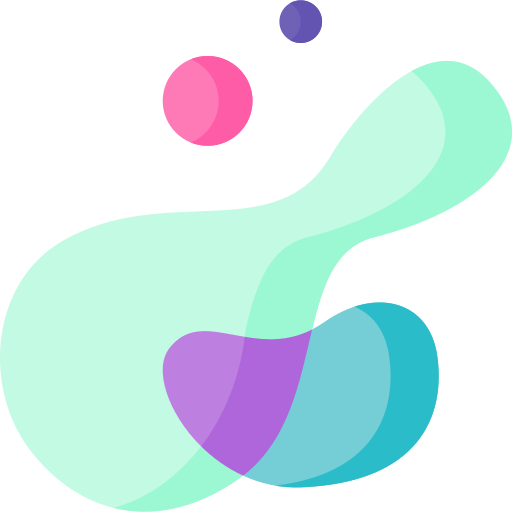
Abstraction
Abstraction is the process of taking away or removing characteristics from something in order to reduce it to a set of essential characteristics.
Learn More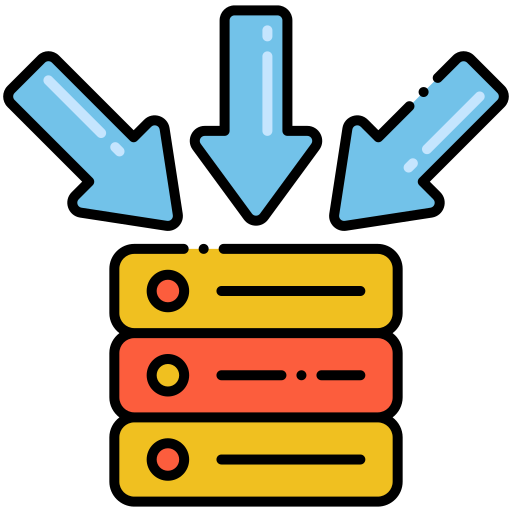
Parameters
A parameter is a variable that is used to pass information into functions . The actual information passed is called an argument.
Learn More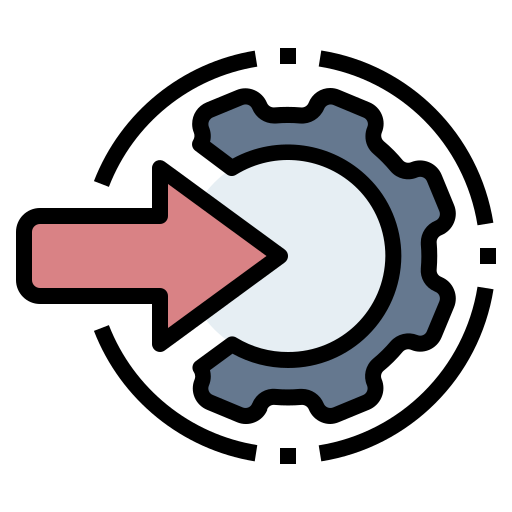
Argument
An argument is a way for you to provide more information to a function. The function can then use that information as it runs, like a variable.
Learn More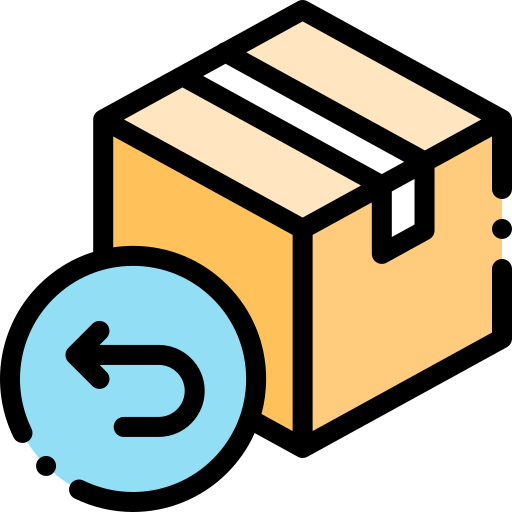
Return Statement
A return statement returns the output of the function and stops running the function. No code runs after the return statement. The call will take on the value of the return.
Learn More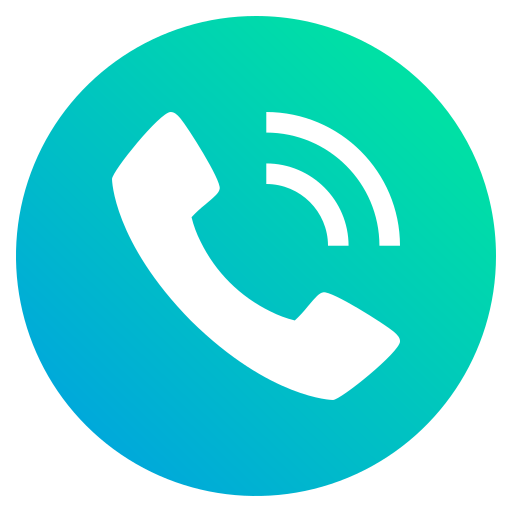
Function Call
A function call means to execute (or run) it. A function is first defined - that is, you write the code it executes and the inputs it needs. Then, when the needed, you call it using its name.
Learn MoreThe S.N.O.T protocol is designed to support students in their problem-solving endeavors. It reinforces the notion that students are capable problem-solvers who can arrive at a solution by following a particular algorithm.
The protocol is designed to develop student engagement, autonomy, and agency as programmers who can address a challenge in a constructive manner.
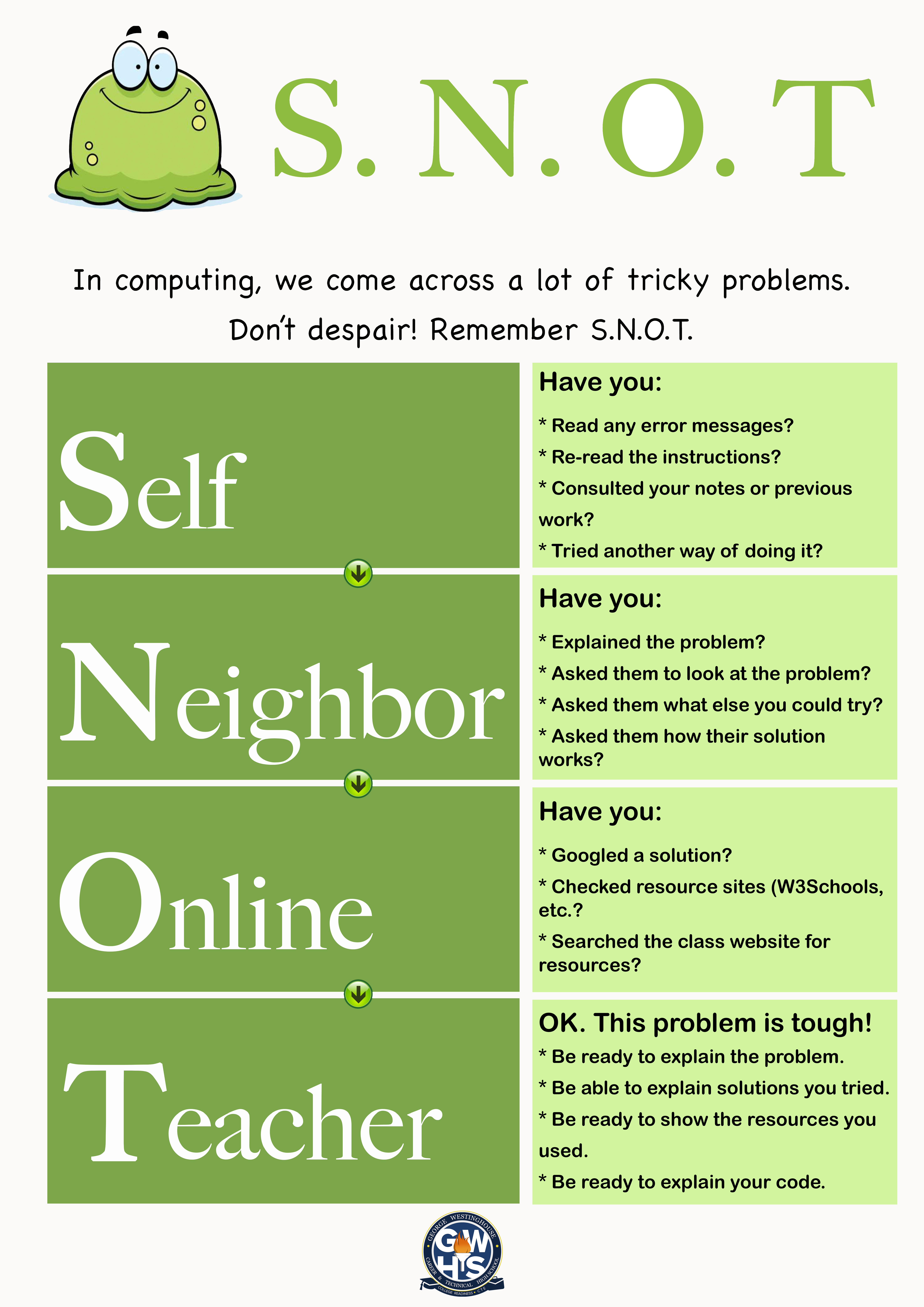
Making notes is an essential aspect of learning in this course. The sources here are designed to support students' ability to extract the salient information from the text-based and visual media they are asked to engage with.
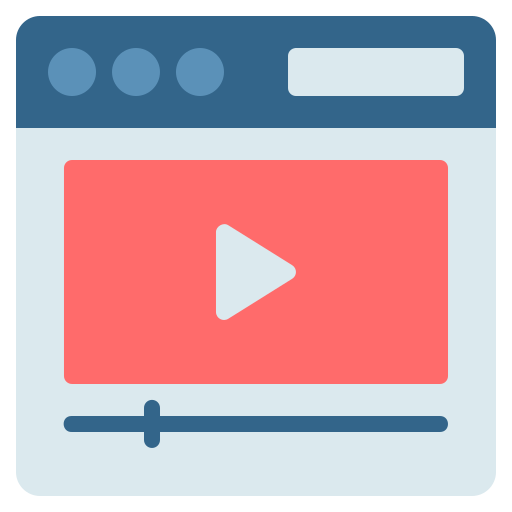
Making Video Notes
Use the resources below to learn about effective and data-informed methods to get the most out of visual media.
Learn More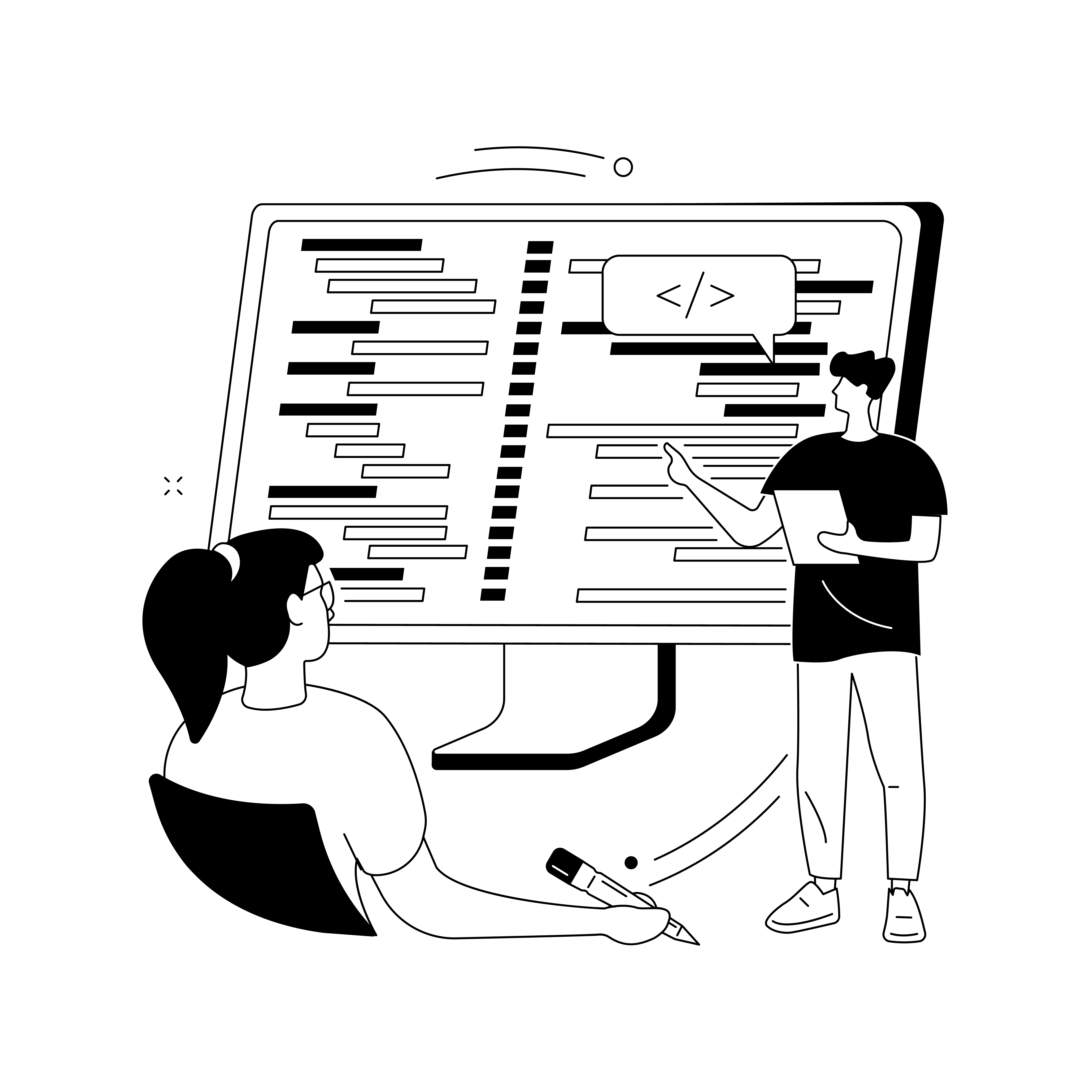
Making Text-Based Notes
Use the resources below to learn about effective and data-informed methods to get the most out of texts.
Learn MoreAt this point, we are exercising much more control over the files we use to support our work. To this end, it may be necessary to import the Bootstrap library into your project in order to take advance of the numerous shortcuts and other benefits it provides.
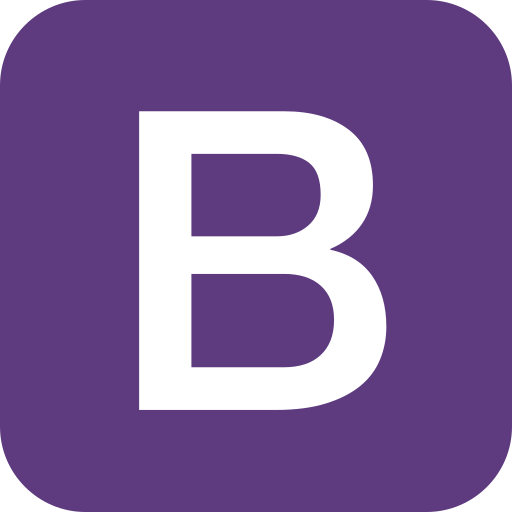
Downloading & Uploading Bootstrap
Use the resources below to learn how to make Bootstrap a part of your project.
Access chatGPTchatGPT is an AI Chatbot developed by Open AI. The chatbot has a language-based model made for interacting with humans.
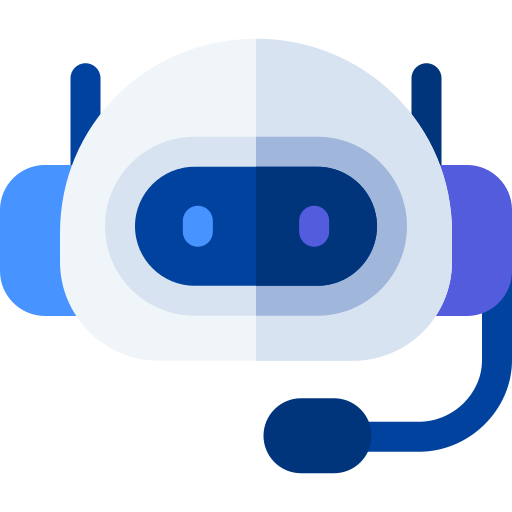
chatGPT
Use the AI chatbot to help you understand content in JavaScript. DO NOT use the AI chatbot to cheat. You may need to use it on your phone for now (may be blocked at school)
Learn MoreNovaApp is an AI Chatbot that uses the chatGPT API to power interaction with humans. The chatbot has a language-based model made for interacting with humans.
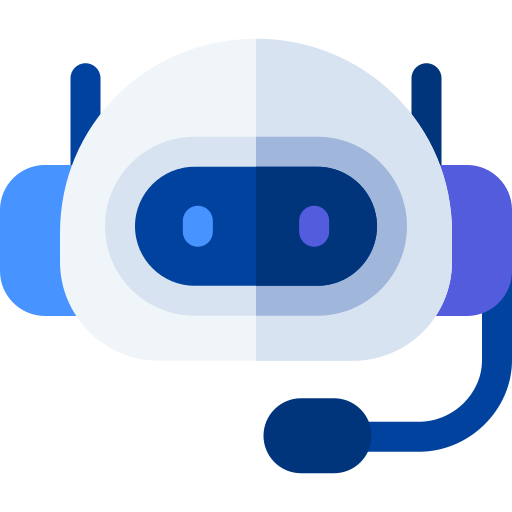
NovaApp
Use the AI chatbot to help you understand content in JavaScript. DO NOT use the AI chatbot to cheat. You may need to use it on your phone for now (may be blocked at school)
Access Nova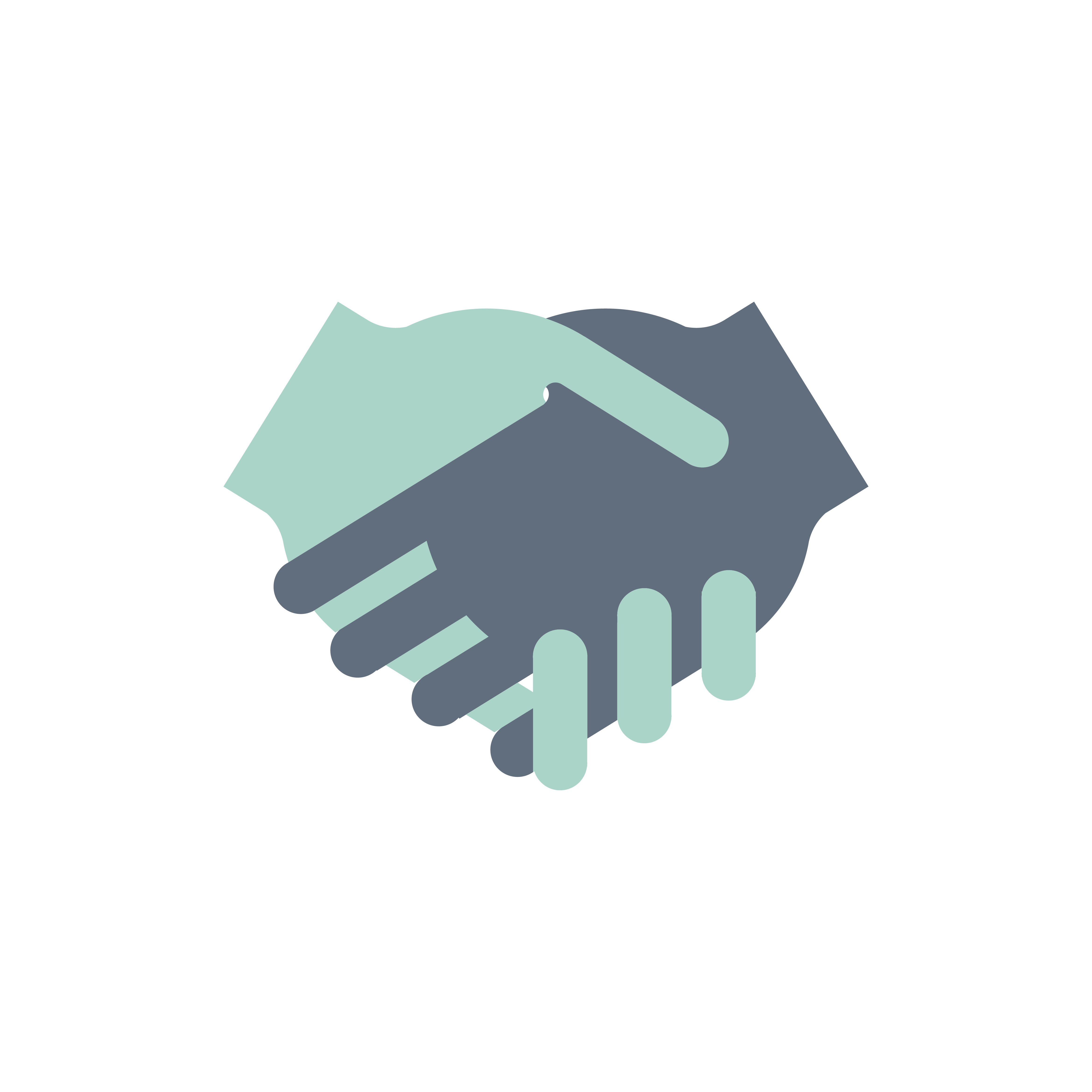
Review & Revise
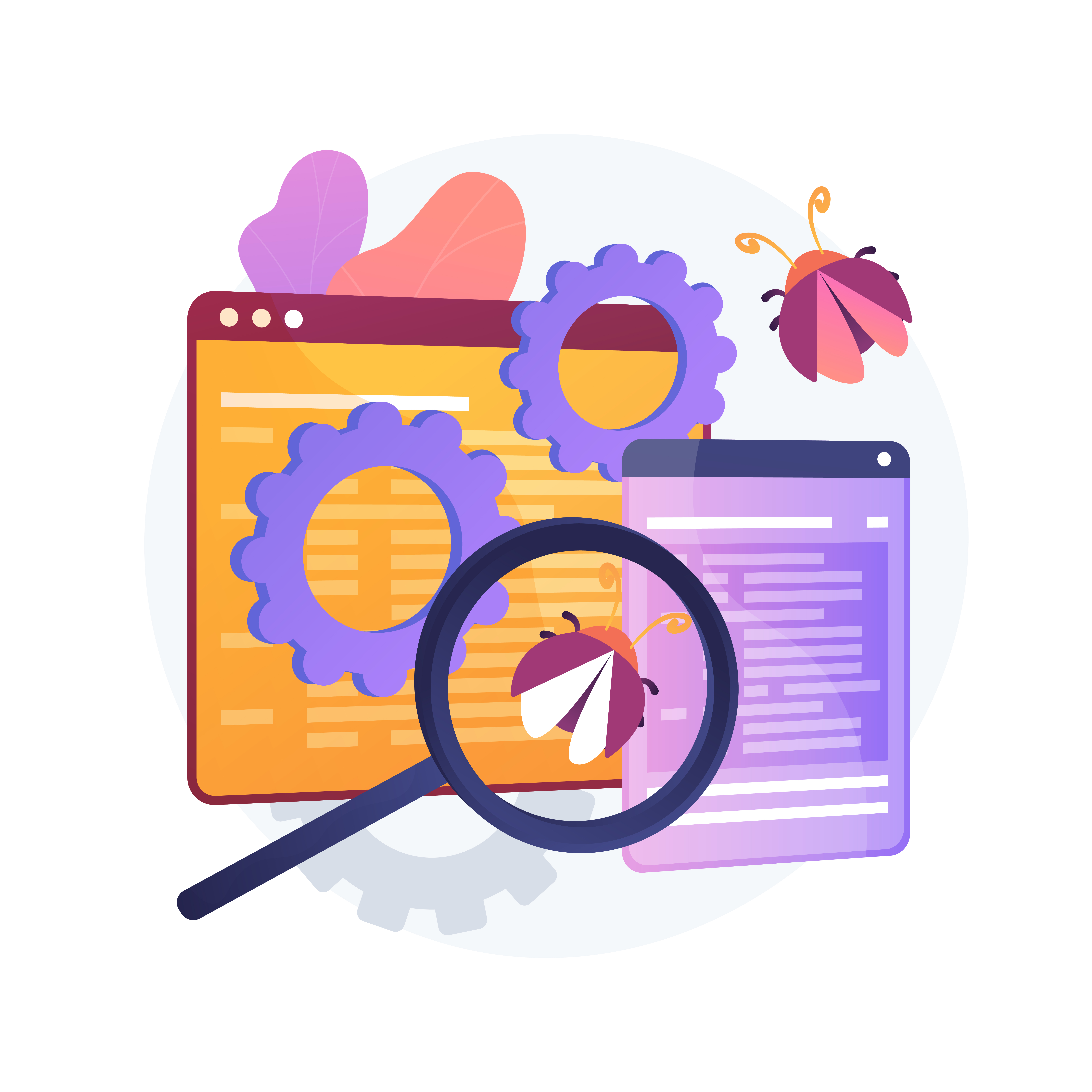
Written Responses
- A. Look over your work section, by section in order to guarantee the highest grade—and a true reflection of your newly gained skills.
- Review your written responses for accuracy and thoroughness
- Next, review your written responses for fluency (making sure the responses represent complete thoughts that fully address the prompt)
- Make any necessary revisions prior to submitting the work.
Code Scripts
- A. Next, you will review your code for readability, efficiency, and elegance.
- Ensure that each task is labeled using comments
- Review your code segments individually and line by line.
- Remove any bugs that may interfere with the program's execution
- Ensure that the scripts you wrote meet the task criteria
- Make sure that your code is highly legible and properly indented
ADDITIONAL DEBUGGING RESOURCES
-
Websites
Here are some more articles to look into...
-
Videos
Here are some additional videos to check out...
Self-Reflection & Evaluation
Self-Evaluation
- A. Let's evaluate our own work based on the assignment rubric. Be as realistic as possible in your evaluation.
- In your U2L2 Workbook, select the "self-evaluation" tab. Check off each criteria based on the level of performance you believe you achieved.
- Add any notes or other communication you wish to convey to your teacher in the appropriate space.
Self-Reflection
- A. Finally, you will reflect on your skills development and ability to complete the lab.
- In your U2L2 Workbook, select the "self-reflection" tab.
- Respond to each prompt using complete sentences that express complete thoughts. Be sure to use the "Where Am I" rubric to determine your performance on certain tasks.
Lab Submission
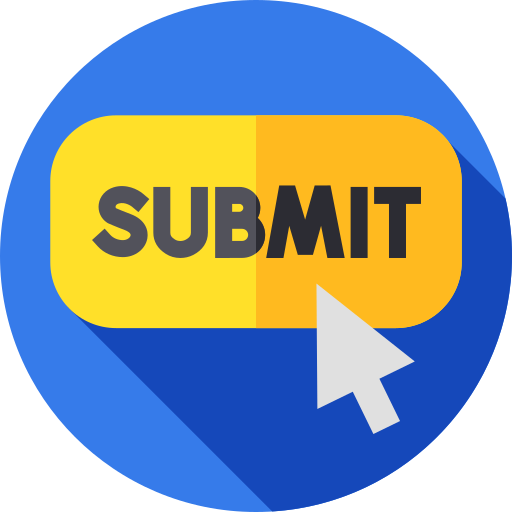
Attach Your Workbook
- A. Let's attach your workbook to Google Classroom. Here's what you need to do:
- Open your U2L2 Workbook.
- Change the share settings on the document to "anyone with the link" and "Editor".
- Copy the link to the newly shared file and paste it in your Google Classroom turn in screen.
VS Code Submission
- A. Now, let's share your project folder so that your JavaScript work can be evaluated. Here's what you need to do:
- Open your project folder (you will need to find where you stored the actual folder on you computer).
- Upload the entire folder to your Google Drive.
- Once the folder has been uploaded to Google Drive, change the share settings on the folder to "anyone with the link" and "Editor".
- Copy the link to the newly shared folder and paste it in your Google Classroom turn in screen.
- Finally, turn in your assignment for evaluation by your teacher.
REPLIT Submission
- A. You can sumbit your work via replit , if you wish. Here's what you need to do:
- If you created your work in replit, simply paste the link to your lab submission in Google Classroom.
- If you created your work in VS Code: find the folder on your computer where the project is stored, upload it to replit and then share that file in your Google Classroom.
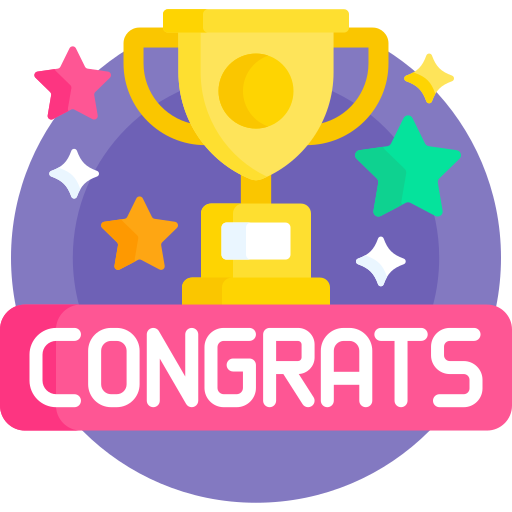